By: Arif Khan.
I assume that you have read my previous article about JavaScript promise, if not then you can read it from here. Always remember before going forward, we should have adequate understanding of previous topics, because there is a relation between each topic.
Our Goal.
In this article we will try to discover JavaScript promise chaining patterns, everyone knows about the chains made from iron rings connected to each other one after another and build a chain. Just like this we can chain our asynchronous operations in sequence or synchronous. Let’s understand by an example, sometimes, we need to execute more than one asynchronous related operations one after another on the basis of first operation results, another operation takes those results and do its operation according to given instructions. Look into below example, the idea is that the result of previous promise passed with .then handler to another promise.
Flow of Chain.
- The vary promise resolves in 1 seconds.
- With .then handler new promise created and resolves with 2 values.
- With another .then handler it takes the returned value from the previous one and after processing, it passes the value to next handler …..and so on.
In this example we can see that the result is passed by the chain of handlers, you can note the alert sequence calls: 1 2 4.

Example Code of promise chain
new Promise(function(resolve, reject) {
setTimeout(() => resolve(1), 1000); // (*)
}).then(function(result) { // (**)
alert(result); // 1
return result * 2;
}).then(function(result) { // (***)
alert(result); // 2
return result * 2;
}).then(function(result) {
alert(result); // 4
return result * 2;
});
You should copy the above code and try it by yourself. Whole promise chain code works well because every call to a .then handler returning a new promise so that you can call the next .then handler. Whenever a handler return the result of the promise, next .then will be called. We can also use multiple .then within a single promise, do not mix it with chaining.
See below example
Here we use multiple handlers in one promise, but they are not shifting the result to new promise. They are executing separately. We seldom use more than one handlers in one promise, but on the other hand promise chaining is often used.
let promise = new Promise(function(resolve, reject) {
setTimeout(() => resolve(1), 1000);
});
promise.then(function(result) {
alert(result); // 1
return result * 2;
});
promise.then(function(result) {
alert(result); // 1
return result * 2;
});
promise.then(function(result) {
alert(result); // 1
return result * 2;
});
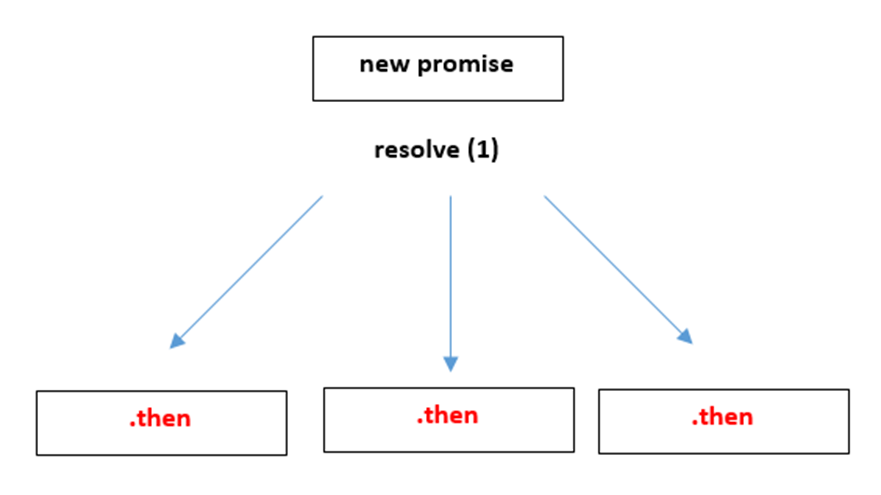
A promise return a promise
We can use a handler within .then to return a promise from a promise .then(handler).
In these situations upcoming handlers wait until its settlement.
e.g.
new Promise(function(resolve, reject) {
setTimeout(() => resolve(1), 1000);
}).then(function(result) {
alert(result);
return new Promise((resolve, reject) => { // R-1
setTimeout(() => resolve(result * 2), 1000);
});
}).then(function(result) { // R-2
alert(result);
return new Promise((resolve, reject) => {
setTimeout(() => resolve(result * 2), 1000);
});
}).then(function(result) {
alert(result); // 4
});
In above example the first .then display 1 and return new promise(…) at the line // R-1. It resolves after one second and the result is transferred to the handler of the 2nd .then. The handler in at line // R-2, it will display 2 and will do the same. The output will be the same as showed in last example. With this method we can build the chain of promises of asynchronous activities.
Share the post with others. If you like.